Calling Server-Side Functions in ServiceNow
Sometimes it is necessary to perform Backend-intensive calculations in a Client UI Script in ServiceNow. Typical example would be the highlighting of fields in a List and Form View upon user-defined conditions. This article will put a simple recipe together, how this can be done with a help of AjaxProcessor.
First step is to create a Script Include, which is going to encapsulate needed condition check. Say, we want to highlight all Agile Stories, where due date is in the past. We create new Script Include with the following simple function getState.
StoryState.prototype = {
getState: function(story) {
if (story.due_date <= gs.endOfToday()) {
return 'red';
}
return 'lightgreen';
},
type: "StoryState"
});
The function returns background color for the story, indicating, whether it is expired or not. At this point we already can create a new Field Style, which will adjust colors in a List View.
Good so far, but how do we highlight expired Stories in a Form View? Field Styles do not apply to Forms. We need to create a Client Script for this. In a Story-Table settings add a new Client Script.
UI Type would be Desktop, Type - onLoad, because we want to check the Conditions once the Form is loaded. Now we can paint our fields on Form with a simple code:
ctrl.style.backgroundColor = 'green';
Now there is only two things left. We would like to call the function of StoryState-Class to determine, which color to apply. The Problem is - this Class is defined on Server-Side and isn't available in Form by default. We need to extend our Class definition and inherit it from AbstractAjaxProcessor.
StoryState.prototype = Object.extendsObject(AbstractAjaxProcessor, {
getState: function (story) {
if (story.due_date <= gs.endOfToday()) {
return 'red';
}
return 'lightgreen';
},
type: "StoryState"
});
There is also an additional function to be added. This function is going to expose the Server-Side functionality to Client Scripts:
getStateAjax: function() {
var storyid = this.getParameter('sysparm_storyid');
var story = new GlideRecord('rm_story');
story.addQuery('sys_id', storyid);
story.query();
story.next();
var res = this.getState(story);
return res;
},
The last step is to call newly exposed Function from Client-Side script. We are using GlideAjax Class for this, which receives three Parameters: Script Include-Class in constructor, Function name as Parameter "sysparm_name" and a sys_id of the validated Story as user-defined parameter.
function onLoad() {
var ga = new GlideAjax('StoryState');
ga.addParam('sysparm_name', 'getStateAjax');
ga.addParam('sysparm_storyid', g_form.getUniqueValue());
ga.getXMLAnswer(callBack);
function callBack(answer) {
var ctrl = g_form.getControl('due_date');
if (!ctrl) return;
ctrl.style.backgroundColor = answer;
}
}
Now if everything have been set up properly, you will see Due Date-Field colored according to Story state. This is a simple example, it can obviously be extended to enable Server-Side processing of any complexity. Have fun!
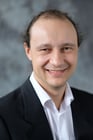